Here is a video tutorial and button trading expert advisor code for MetaTrader 4 platform. You can use the expert advisor to send automated orders to the Algomojo Platform with just the click of a button.
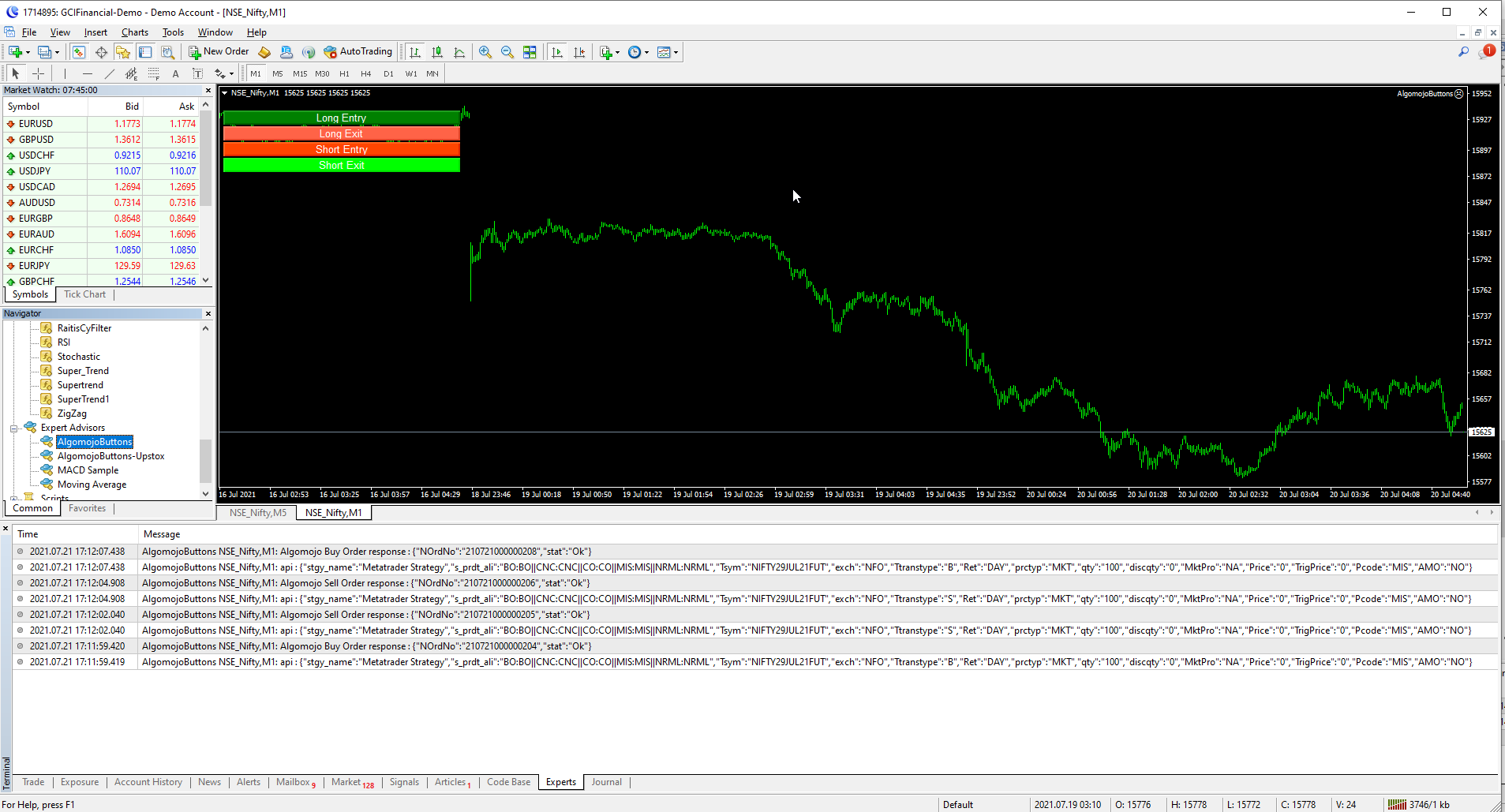
How to Send Automated Orders to Metatrader 4 Platform
You have to follow the 6 steps to send automated orders
Step 1: Make sure you have a trading account with Algomojo Partner Broker. If not get one. Login to your Algomojo Partner Broker Account. Currently, Algmojo supports Aliceblue, Tradejini, Zebu & Mastertrust.
Step 2. Download and Install the Multi Broker Bridge
Step 3: Download the Algomojo Expert Advisor and Copy the Expert Advisor File AlgomojoButtons.ex4
Step 4: Open your Metatader 4 Platform and Goto File ->Open Data Folder->Open MQL4/Experts Folder->Paste the AlgomojoButtons.ex4 as shown below
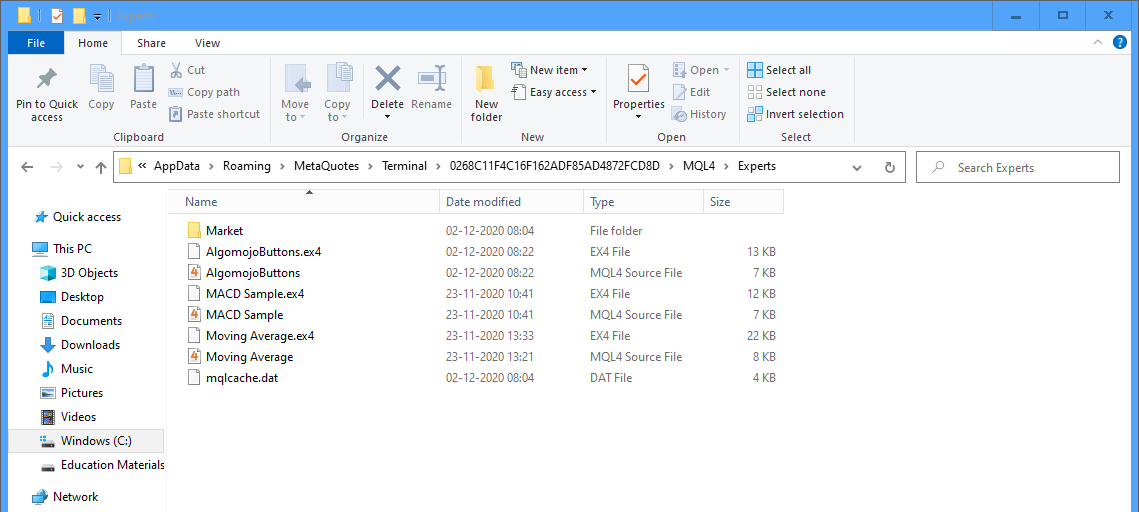
Step 5: Configuring the Algomojo Expert Advisor
i)Drag and drop the Expert Advisor to the charts
ii)Enable Allow DLL Imports from the common menu of Expert Advisor Properties
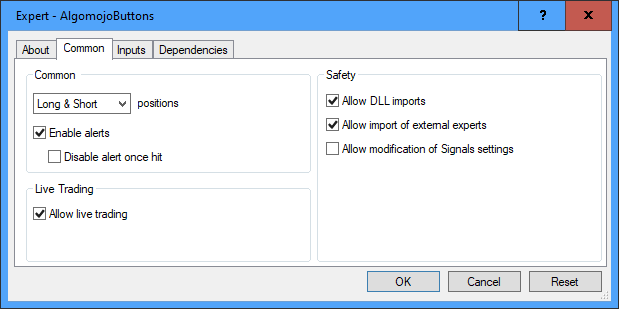
iii)Set the API key and API Secret key that you receive from the Algomojo My API section . Now set the appropriate exchange (NSE,NFO, MCX..etc) and select the appropriate symbol, order details , enter the broker shortcode and API version and Enable_Algo = True and Press the OK Button
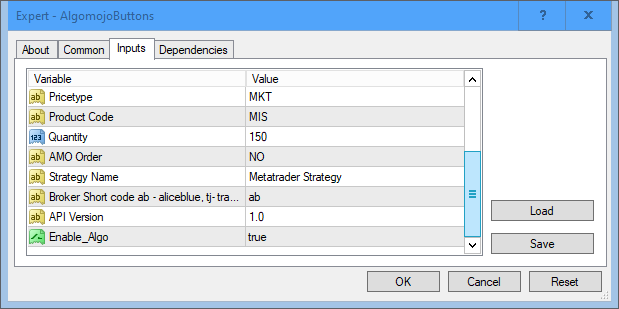
Step 6 : Press the Buy or Sell Button to send Automated orders from Metatrader 4 to MQL4 trading terminal and check out the same in the expert advisor log section

Watch this video tutorial to configure AlgmojoButtons Expert Advisor and Send Automated Orders to your broker account.
In case if you need any assistance kindly check with Algomojo Tech support team or send a email at support@algomojo.com
Download Algomojo MQL4 code
//+------------------------------------------------------------------+
//| AlgomojoButtons.mq4 |
//| Copyright 2020, Marketcalls Financial Services Pvt Ltd |
//| https://www.marketcalls.in |
//+------------------------------------------------------------------+
#property copyright "Copyright 2020, Marketcalls Financial Services Pvt Ltd"
#property link "https://www.marketcalls.in"
#property version "1.00"
#property strict
//Algomojo Autotrading Modules
input string user_apikey = "xxxxxxxxxxxxxxxxxxxxxx"; //Enter your API key here
input string api_secretkey = "xxxxxxxxxxxxxxxxxxxxxx"; //Enter your API secret key here
input string s_prdt_ali = "BO:BO||CNC:CNC||CO:CO||MIS:MIS||NRML:NRML";
input string Tsym = "CRUDEOIL21FEBFUT"; //Symbol Name
input string exch = "MCX"; //Exchange
input string Ret = "DAY"; //Retention
input string prctyp = "MKT"; // Pricetype
input string Pcode = "MIS"; // Product Code
input int qty = 100; // Quantity
input string AMO = "NO"; //AMO Order
input string stgy_name = "Metatrader Strategy"; // Strategy Name
input string broker = "ab"; //Broker Short code ab - aliceblue, tj- tradejini, zb - zebu , en - enrich
input string ver = "1.0"; //API Version
string response;
string api_data;
#import "AMMT4BRIDGE.dll"
string AMDispatcher(string api_key, string api_secret, string api_name, string api_data, string br_code, string version);
#import
extern bool Enable_Algo = false;
//+------------------------------------------------------------------+
//| Expert initialization function |
//+------------------------------------------------------------------+
int OnInit()
{
//if(IsTesting())
{
string name;
string heading[4]={"Long Entry","Long Exit","Short Entry","Short Exit"};
int xc=5;
int yc=30;
int xsize = 500;
int ysize = 300;
for(int i=0;i<4;i++)
{
name=heading[i];
ObjectCreate(0,name,OBJ_BUTTON,0,0,0);
ObjectSetText(name,name,16,"Arial",clrWhite);
ObjectSetInteger(0,name,OBJPROP_XSIZE,xsize);
ObjectSetInteger(0,name,OBJPROP_XSIZE,ysize);
ObjectSetInteger(0,name,OBJPROP_XDISTANCE,xc);
ObjectSetInteger(0,name,OBJPROP_YDISTANCE,yc);
ObjectSetInteger(0,name,OBJPROP_COLOR,clrWhite);
if(i==0)
{
ObjectSetInteger(0,name,OBJPROP_BGCOLOR,clrGreen);
}
if(i==1)
{
ObjectSetInteger(0,name,OBJPROP_BGCOLOR,clrTomato);
}
if(i==2)
{
ObjectSetInteger(0,name,OBJPROP_BGCOLOR,clrOrangeRed);
}
if(i==3)
{
ObjectSetInteger(0,name,OBJPROP_BGCOLOR,clrLime);
}
yc+=20;
}
}
//---
return(INIT_SUCCEEDED);
}
//+------------------------------------------------------------------+
//| Expert deinitialization function |
//+------------------------------------------------------------------+
void OnDeinit(const int reason)
{
//Delete the Buttons
string ButtonName;
string heading[4]={"Long Entry","Long Exit","Short Entry","Short Exit"};
for(int i=0;i<4;i++)
{
ButtonName=heading[i];
ObjectDelete(ButtonName);
}
//---
}
//+------------------------------------------------------------------+
//| Expert tick function |
//+------------------------------------------------------------------+
void OnTick()
{
//---
}
//+------------------------------------------------------------------+
//| BuyOrder function |
//+------------------------------------------------------------------+
void BuyOrder()
{
//Algomojo Place Buy Order
api_data ="{\"stgy_name\":\""+stgy_name+"\",\"s_prdt_ali\":\""+s_prdt_ali+"\",\"Tsym\":\""+Tsym+"\",\"exch\":\""+exch+"\",\"Ttranstype\":\""+"B"+"\",\"Ret\":\""+Ret+"\",\"prctyp\":\""+prctyp+"\",\"qty\":\""+qty+"\",\"discqty\":\""+"0"+"\",\"MktPro\":\""+"NA"+"\",\"Price\":\""+"0"+"\",\"TrigPrice\":\""+"0"+"\",\"Pcode\":\""+Pcode+"\",\"AMO\":\""+AMO+"\"}";
response=AMDispatcher(user_apikey, api_secretkey,"PlaceOrder",api_data,broker,ver);
Print("api : " ,api_data);
Print("Algomojo Buy Order response : " ,response);
}
//+------------------------------------------------------------------+
//| SellOrder function |
//+------------------------------------------------------------------+
void SellOrder()
{
//Algomojo Place Sell Order
api_data ="{\"stgy_name\":\""+stgy_name+"\",\"s_prdt_ali\":\""+s_prdt_ali+"\",\"Tsym\":\""+Tsym+"\",\"exch\":\""+exch+"\",\"Ttranstype\":\""+"S"+"\",\"Ret\":\""+Ret+"\",\"prctyp\":\""+prctyp+"\",\"qty\":\""+qty+"\",\"discqty\":\""+"0"+"\",\"MktPro\":\""+"NA"+"\",\"Price\":\""+"0"+"\",\"TrigPrice\":\""+"0"+"\",\"Pcode\":\""+Pcode+"\",\"AMO\":\""+AMO+"\"}";
response=AMDispatcher(user_apikey, api_secretkey,"PlaceOrder",api_data,broker,ver);
Print("api : " ,api_data);
Print("Algomojo Sell Order response : " ,response);
}
//+------------------------------------------------------------------+
//| ChartEvent function |
//+------------------------------------------------------------------+
void OnChartEvent(const int id,
const long &lparam,
const double &dparam,
const string &sparam)
{
if(id==CHARTEVENT_OBJECT_CLICK)
{
if(ObjectGetInteger(0,"Long Entry",OBJPROP_STATE)==true)
{
if(Enable_Algo){
//Algomojo Place Buy Order - Double the Quantity
ObjectSetInteger(0,"Long Entry",OBJPROP_STATE,false);
BuyOrder();
}
}
if(ObjectGetInteger(0,"Short Entry",OBJPROP_STATE)==true)
{
if(Enable_Algo){
//Algomojo Place Buy Order - Double the Quantity
ObjectSetInteger(0,"Short Entry",OBJPROP_STATE,false);
SellOrder();
}
}
if(ObjectGetInteger(0,"Long Exit",OBJPROP_STATE)==true)
{
if(Enable_Algo){
//Algomojo Place Buy Order - Double the Quantity
ObjectSetInteger(0,"Long Exit",OBJPROP_STATE,false);
SellOrder();
}
}
if(ObjectGetInteger(0,"Short Exit",OBJPROP_STATE)==true)
{
if(Enable_Algo){
//Algomojo Place Buy Order - Double the Quantity
ObjectSetInteger(0,"Short Exit",OBJPROP_STATE,false);
BuyOrder();
}
}
}
//---
}
hello,
can you please create mt5 buttons also?
Let me check the possibility and will create it.
Hi
I am getting below error , please advise.
2021.12.31 19:56:13.812 Cannot find ‘AMDispatcher’ in ‘AMMT4BRIDGE.dll’
Kindly get in touch with the support team. They will be able to fix your issue.
Getting following Error
2022.03.04 12:52:46.751 AlgomojoButtons NIFTY#,M5: unresolved import function call
2022.03.04 12:52:46.751 Cannot find ‘AMDispatcher’ in ‘AMMT4BRIDGE.dll’
Kindly connect with our chat support team. They will be able to assist on implementing the bridge.
Is it possible to place a limit order rather than market order using the Algomojo button?