Got frequent requirements from a couple of traders to automate Excel based button trading where with a click of a button one could punch orders in multiple accounts (friends & family).
Designed a simple interface in excel on sending simultaneous orders from the excel application to multiple trading accounts. Code uses algomojo API to transmit multiple orders using the PlaceMultiOrder function.
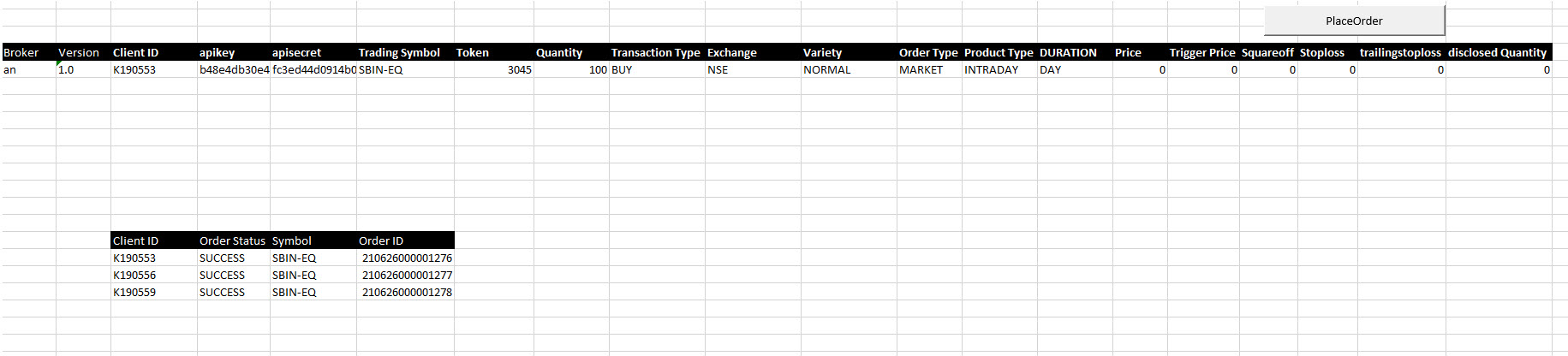
Supported Broker: Angel Angel Broking, AliceBlue, Firstock, Tradejini, Mastertrust, Zebu
Requirements: Algomojo Login
Steps to follow after downloading the Excel File
1)Download the File and Unzip it to the local folder
2)Press Enable Content button as the Security Warning display that Macros have been disabled
3)Sheet Place Order is used to Send MultiOrders
4)Sheet Clients is used to manage multiple clients, API Keys , API Secret Keys
Here are the Angel Broking supported order parameters in the excel sheets
Param | Value | Description |
---|---|---|
variety | NORMAL STOPLOSS AMO ROBO | Normal Order (Regular) Stop-loss order, After Market Order, ROBO (Bracket Order) |
transactiontype | BUY SELL | Buy Sell |
ordertype | MARKET LIMIT STOPLOSS_LIMIT STOPLOSS_MARKET | Market Order(MKT) Limit Order(L) Stop Loss Limit Order(SL) Stop Loss Market Order(SL-M) |
producttype | DELIVERY CARRYFORWARD MARGIN INTRADAY BO | Cash & Carry for equity (CNC) Normal for futures and options (NRML) Margin Delivery Margin Intraday Square off (MIS) Bracket Order (Only for ROBO) |
Duration | DAY IOC | Regular Order Immediate or Cancel |
exchange | BSE NSE NFO MCX | BSE Equity NSE Equity NSE Future and Options MCX Commodity |
Order Parameters
These parameters are common across different order varieties.
Param | Description |
---|---|
tradingsymbol | Trading Symbol of the instrument |
symboltoken | Symbol Token is unique identifier ( can be obtained from Algomojo Watchlist section->Security Info) |
Exchange | Name of the exchange (BSE,NSE,NFO,MCX) |
transactiontype | BUY or SELL |
ordertype | Order type (MARKET, LIMIT etc.) |
quantity | Quantity to transact |
producttype | Product type (CNC,MIS) |
price | The min or max price to execute the order at (for LIMIT orders) |
triggerprice | The price at which an order should be triggered (SL, SL-M) |
squareoff | Only For ROBO (Bracket Order) |
stoploss | Only For ROBO (Bracket Order) |
trailingStopLoss | Only For ROBO (Bracket Order) |
disclosedquantity | Quantity to disclose publicly (for equity trades) |
duration | Order duration (DAY,IOC) |
VBA Source Code for Angel Broking
Sub FetchLogin()
Dim Broker As String
Dim Version As String
Dim apikey As String
Dim apisecret As String
Dim apidata As String
Dim numofRows As Integer
Dim i As Integer
Broker = "an"
Version = "1.0"
numofRows = Range("A5", Range("A5").End(xlDown)).Rows.Count
apidata = "{}"
For i = 0 To numofRows - 1
apikey = Sheets("Clients").Cells(5 + i, "B").Value
apisecret = Sheets("Clients").Cells(5 + i, "C").Value
Limits = AMConnect(apikey, apisecret, Broker, Version, "Limits", apidata)
Profile = AMConnect(apikey, apisecret, Broker, Version, "Profile", apidata)
Set ProfileResponse = JsonConverter.ParseJson(Profile)
Set LimitsResponse = JsonConverter.ParseJson(Limits)
If StrComp(ProfileResponse("message"), "SUCCESS") = 0 Then
Sheets("Clients").Cells(5 + i, "E") = ProfileResponse("data")("name")
Sheets("Clients").Cells(5 + i, "G") = LimitsResponse("data")("net")
Sheets("Clients").Cells(5 + i, "G") = LimitsResponse("data")("availablecash")
Else
Sheets("Clients").Cells(5 + i, "E") = "Login Error"
Sheets("Clients").Cells(5 + i, "F") = "Login Error"
Sheets("Clients").Cells(5 + i, "G") = "Login Error"
End If
Next
End Sub
Sub AlgomojoMulitOrder()
Dim Broker As String
Dim Version As String
Dim ClientID As String
Dim user_apikey As String
Dim api_secret As String
Dim masterapikey As String
Dim masterapisecret As String
Dim variety As String
Dim tradingsymbol As String
Dim symboltoken As String
Dim transactiontype As String
Dim exchange As String
Dim ordertype As String
Dim producttype As String
Dim duration As String
Dim price As String
Dim squareoff As String
Dim stoploss As String
Dim quantity As String
Dim triggerprice As String
Dim trailingStopLoss As String
Dim disclosedquantity As String
Dim MultiplierQty As Long
Dim apidata As String
Dim numofRows As Long
Dim i As Integer
Broker = Sheets("PlaceOrder").Cells(5, "A").Value
Version = Sheets("PlaceOrder").Cells(5, "B").Value
masterapikey = Sheets("PlaceOrder").Cells(5, "D").Value
masterapisecret = Sheets("PlaceOrder").Cells(5, "E").Value
tradingsymbol = Sheets("PlaceOrder").Cells(5, "F").Value
symboltoken = Sheets("PlaceOrder").Cells(5, "G").Value
quantity = Sheets("PlaceOrder").Cells(5, "H").Value
transactiontype = Sheets("PlaceOrder").Cells(5, "I").Value
exchange = Sheets("PlaceOrder").Cells(5, "J").Value
variety = Sheets("PlaceOrder").Cells(5, "K").Value
ordertype = Sheets("PlaceOrder").Cells(5, "L").Value
producttype = Sheets("PlaceOrder").Cells(5, "M").Value
duration = Sheets("PlaceOrder").Cells(5, "N").Value
price = Sheets("PlaceOrder").Cells(5, "O").Value
triggerprice = Sheets("PlaceOrder").Cells(5, "P").Value
squareoff = Sheets("PlaceOrder").Cells(5, "Q").Value
stoploss = Sheets("PlaceOrder").Cells(5, "R").Value
trailingStopLoss = Sheets("PlaceOrder").Cells(5, "S").Value
disclosedquantity = Sheets("PlaceOrder").Cells(5, "T").Value
'clear the table contents of the display output
Worksheets("PlaceOrder").Range("C16:F200").Clear
Sheets("Clients").Activate
numofRows = Sheets("Clients").Range("A5", Range("A5").End(xlDown)).Rows.Count
apidata = "{""" & "orders" & """:" & "[ "
stgy_name = "Excel"
For i = 0 To numofRows - 1
Sheets("Clients").Activate
ClientID = Sheets("Clients").Cells(5 + i, "A").Value
user_apikey = Sheets("Clients").Cells(5 + i, "B").Value
api_secret = Sheets("Clients").Cells(5 + i, "C").Value
MultiplierQty = Sheets("Clients").Cells(5 + i, "D").Value * CInt(quantity)
Sheets("PlaceOrder").Activate
Sheets("PlaceOrder").Cells(16 + i, "C") = ClientID
apidata = apidata & "{""" _
& "order_refno" & """:""" & CStr(i + 1) & """,""" _
& "user_apikey" & """:""" & user_apikey & """,""" _
& "api_secret" & """:""" & api_secret & """,""" _
& "stgy_name" & """:""" & stgy_name & """,""" _
& "variety" & """:""" & variety & """,""" _
& "tradingsymbol" & """:""" & tradingsymbol & """,""" _
& "symboltoken" & """:""" & symboltoken & """,""" _
& "transactiontype" & """:""" & transactiontype & """,""" _
& "exchange" & """:""" & exchange & """,""" _
& "ordertype" & """:""" & ordertype & """,""" _
& "producttype" & """:""" & producttype & """,""" _
& "duration" & """:""" & duration & """,""" _
& "price" & """:""" & price & """,""" _
& "squareoff" & """:""" & squareoff & """,""" _
& "stoploss" & """:""" & stoploss & """,""" _
& "quantity" & """:""" & CStr(MultiplierQty) & """,""" _
& "triggerprice" & """:""" & triggerprice & """,""" _
& "trailingStopLoss" & """:""" & trailingStopLoss & """,""" _
& "disclosedquantity" & """:""" & disclosedquantity
If i = numofRows - 1 Then
apidata = apidata & """}"
Else
apidata = apidata & """},"
End If
Next
apidata = apidata & "]}"
PlaceOrder = AMConnect(masterapikey, masterapisecret, Broker, Version, "PlaceMultiOrder", apidata)
Set PlaceOrderResponse = JsonConverter.ParseJson(PlaceOrder)
For i = 0 To numofRows - 1
If StrComp(PlaceOrderResponse(i + 1)("message"), "SUCCESS") = 0 Then
Sheets("PlaceOrder").Cells(16 + i, "D").Value = PlaceOrderResponse(i + 1)("message")
Sheets("PlaceOrder").Cells(16 + i, "E").Value = PlaceOrderResponse(i + 1)("data")("script")
Sheets("PlaceOrder").Cells(16 + i, "F").Value = PlaceOrderResponse(i + 1)("data")("orderid")
Sheets("PlaceOrder").Cells(16 + i, "F").NumberFormat = "0"
Else
Sheets("PlaceOrder").Cells(16 + i, "D") = "Login Error"
Sheets("PlaceOrder").Cells(16 + i, "E") = "Login Error"
Sheets("PlaceOrder").Cells(16 + i, "F") = "Login Error"
End If
Next
'check multiorder response
'Sheets("PlaceOrder").Cells(12, "D") = PlaceOrder
End Sub
Private Function AMConnect(api_key As String, api_secret As String, Broker As String, Version As String, api_name As String, InTD As String) As String
Dim objHTTP As Object
Dim result As String
Dim postdate As String
Dim BrkPrefix As String
Dim BaseURL As String
BaseURL = "https://" & LCase(Broker) & "api.algomojo.com/" & Version & "/"
postdata = "{""" & "api_key" & """:""" & api_key & """,""" _
& "api_secret" & """:""" & api_secret & """,""" _
& "data" & """:" & InTD & "}"
'MsgBox (postdata)
Set objHTTP = CreateObject("MSXML2.ServerXMLHTTP")
Url = BaseURL & api_name
objHTTP.Open "POST", Url, False
objHTTP.setRequestHeader "Content-type", "application/json"
objHTTP.send (postdata)
result = objHTTP.responseText
Set objHTTP = Nothing
AMConnect = result
End Function
VBA Source Code for Aliceblue, Zebu, Tradejini, Mastertrust
Sub FetchLogin()
Dim Broker As String
Dim Version As String
Dim uid As String
Dim apikey As String
Dim apisecret As String
Dim apidata As String
Dim segment As String
Dim Exchange As String
Dim product As String
Dim numofRows As Integer
Dim i As Integer
Version = "1.0"
segment = "ALL"
Exchange = ""
product = ""
numofRows = Range("A5", Range("A5").End(xlDown)).Rows.Count
For i = 0 To numofRows - 1
Broker = Sheets("Clients").Cells(5 + i, "A").Value
uid = Sheets("Clients").Cells(5 + i, "B").Value
apikey = Sheets("Clients").Cells(5 + i, "C").Value
apisecret = Sheets("Clients").Cells(5 + i, "D").Value
apidata = "{""" _
& "uid" & """:""" & uid & """,""" _
& "actid" & """:""" & uid & """,""" _
& "segment" & """:""" & segment & """,""" _
& "Exchange" & """:""" & Exchange & """,""" _
& "product" & """:""" & product & """" _
& "}"
Limits = AMConnect(apikey, apisecret, Broker, Version, "Limits", apidata)
'MsgBox (Limits)
Set LimitsResponse = JsonConverter.ParseJson(Limits)
If StrComp(LimitsResponse("stat"), "Ok") = 0 Then
Sheets("Clients").Cells(5 + i, "F") = LimitsResponse("OpeningBalance")
Sheets("Clients").Cells(5 + i, "G") = LimitsResponse("Netcashavailable")
Sheets("Clients").Cells(5 + i, "H") = LimitsResponse("turnover")
Else
Sheets("Clients").Cells(5 + i, "F") = "Login Error"
Sheets("Clients").Cells(5 + i, "G") = "Login Error"
Sheets("Clients").Cells(5 + i, "H") = "Login Error"
End If
Next
End Sub
Sub AlgomojoMulitOrder()
Dim Broker As String
Dim Version As String
Dim ClientID As String
Dim user_apikey As String
Dim api_secret As String
Dim masterapikey As String
Dim masterapisecret As String
Dim s_prdt_ali As String
Dim Tsym As String
Dim exch As String
Dim Ttranstype As String
Dim Ret As String
Dim prctyp As String
Dim qty As String
Dim discqty As String
Dim MktPro As String
Dim Price As String
Dim TrigPrice As String
Dim Pcode As String
Dim AMO As String
Dim MultiplierQty As Long
Dim apidata As String
Dim numofRows As Long
Dim i As Integer
s_prdt_ali = "BO:BO||CNC:CNC||CO:CO||MIS:MIS||NRML:NRML"
MktPro = "NA"
discqty = "0"
AMO = "NO"
Broker = Sheets("PlaceOrder").Cells(5, "A").Value
Version = Sheets("PlaceOrder").Cells(5, "B").Value
masterapikey = Sheets("PlaceOrder").Cells(5, "D").Value
masterapisecret = Sheets("PlaceOrder").Cells(5, "E").Value
Tsym = Sheets("PlaceOrder").Cells(5, "F").Value
qty = Sheets("PlaceOrder").Cells(5, "G").Value
Ttranstype = Sheets("PlaceOrder").Cells(5, "H").Value
exch = Sheets("PlaceOrder").Cells(5, "I").Value
Pcode = Sheets("PlaceOrder").Cells(5, "J").Value
prctyp = Sheets("PlaceOrder").Cells(5, "K").Value
Ret = Sheets("PlaceOrder").Cells(5, "L").Value
Price = Sheets("PlaceOrder").Cells(5, "M").Value
TrigPrice = Sheets("PlaceOrder").Cells(5, "N").Value
discqty = Sheets("PlaceOrder").Cells(5, "O").Value
'clear the table contents of the display output
Worksheets("PlaceOrder").Range("C16:F200").Clear
Sheets("Clients").Activate
numofRows = Sheets("Clients").Range("A5", Range("A5").End(xlDown)).Rows.Count
apidata = "{""" & "orders" & """:" & "[ "
stgy_name = "Excel"
For i = 0 To numofRows - 1
Sheets("Clients").Activate
ClientID = Sheets("Clients").Cells(5 + i, "B").Value
user_apikey = Sheets("Clients").Cells(5 + i, "C").Value
api_secret = Sheets("Clients").Cells(5 + i, "D").Value
MultiplierQty = Sheets("Clients").Cells(5 + i, "E").Value * CInt(qty)
Sheets("PlaceOrder").Activate
Sheets("PlaceOrder").Cells(16 + i, "C") = ClientID
apidata = apidata & "{""" _
& "order_refno" & """:""" & CStr(i + 1) & """,""" _
& "user_apikey" & """:""" & user_apikey & """,""" _
& "api_secret" & """:""" & api_secret & """,""" _
& "stgy_name" & """:""" & stgy_name & """,""" _
& "s_prdt_ali" & """:""" & s_prdt_ali & """,""" _
& "Tsym" & """:""" & Tsym & """,""" _
& "exch" & """:""" & exch & """,""" _
& "exchange" & """:""" & Exchange & """,""" _
& "Ttranstype" & """:""" & Ttranstype & """,""" _
& "Ret" & """:""" & Ret & """,""" _
& "prctyp" & """:""" & prctyp & """,""" _
& "qty" & """:""" & CStr(MultiplierQty) & """,""" _
& "discqty" & """:""" & discqty & """,""" _
& "MktPro" & """:""" & MktPro & """,""" _
& "Price" & """:""" & Price & """,""" _
& "TrigPrice" & """:""" & TrigPrice & """,""" _
& "Pcode" & """:""" & Pcode & """,""" _
& "AMO" & """:""" & AMO
If i = numofRows - 1 Then
apidata = apidata & """}"
Else
apidata = apidata & """},"
End If
Next
apidata = apidata & "]}"
PlaceOrder = AMConnect(masterapikey, masterapisecret, Broker, Version, "PlaceMultiOrder", apidata)
Set PlaceOrderResponse = JsonConverter.ParseJson(PlaceOrder)
For i = 0 To numofRows - 1
If StrComp(PlaceOrderResponse(i + 1)("stat"), "Ok") = 0 Then
Sheets("PlaceOrder").Cells(16 + i, "D").Value = PlaceOrderResponse(i + 1)("stat")
Sheets("PlaceOrder").Cells(16 + i, "E").Value = Tsym
Sheets("PlaceOrder").Cells(16 + i, "F").Value = PlaceOrderResponse(i + 1)("NOrdNo")
Sheets("PlaceOrder").Cells(16 + i, "F").NumberFormat = "0"
Else
Sheets("PlaceOrder").Cells(16 + i, "D") = "Login Error"
Sheets("PlaceOrder").Cells(16 + i, "E") = "Login Error"
Sheets("PlaceOrder").Cells(16 + i, "F") = "Login Error"
End If
Next
'check multiorder response
'Sheets("PlaceOrder").Cells(12, "D") = PlaceOrder
End Sub
Private Function AMConnect(api_key As String, api_secret As String, Broker As String, Version As String, api_name As String, InTD As String) As String
Dim objHTTP As Object
Dim result As String
Dim postdate As String
Dim BrkPrefix As String
Dim BaseURL As String
BaseURL = "https://" & LCase(Broker) & "api.algomojo.com/" & Version & "/"
postdata = "{""" & "api_key" & """:""" & api_key & """,""" _
& "api_secret" & """:""" & api_secret & """,""" _
& "data" & """:" & InTD & "}"
'MsgBox (postdata)
Set objHTTP = CreateObject("MSXML2.ServerXMLHTTP")
Url = BaseURL & api_name
objHTTP.Open "POST", Url, False
objHTTP.setRequestHeader "Content-type", "application/json"
objHTTP.send (postdata)
result = objHTTP.responseText
Set objHTTP = Nothing
AMConnect = result
End Function
VBA Code for Firstock
Sub FetchLogin()
Dim Broker As String
Dim Version As String
Dim uid As String
Dim apikey As String
Dim apisecret As String
Dim apidata As String
Dim segment As String
Dim Exchange As String
Dim product As String
Dim numofRows As Integer
Dim i As Long
Dim Total As Long
Version = "1.0"
numofRows = Range("A5", Range("A5").End(xlDown)).Rows.Count
If numofRows = 1 Then
Total = numofRows
Else
Total = numofRows - 1
End If
For i = 0 To Total
Broker = Sheets("Clients").Cells(5 + i, "A").Value
uid = Sheets("Clients").Cells(5 + i, "B").Value
apikey = Sheets("Clients").Cells(5 + i, "C").Value
apisecret = Sheets("Clients").Cells(5 + i, "D").Value
apidata = "{""" _
& "uid" & """:""" & uid & """,""" _
& "actid" & """:""" & uid & """" & "}"
'MsgBox (apidata)
Limits = AMConnect(apikey, apisecret, Broker, Version, "Limits", apidata)
Set LimitsResponse = JsonConverter.ParseJson(Limits)
If StrComp(LimitsResponse("stat"), "Ok") = 0 Then
Sheets("Clients").Cells(5 + i, "F") = LimitsResponse("cash")
Sheets("Clients").Cells(5 + i, "G") = LimitsResponse("daycash")
Sheets("Clients").Cells(5 + i, "H") = LimitsResponse("brkcollamt")
Else
Sheets("Clients").Cells(5 + i, "F") = "Login Error"
Sheets("Clients").Cells(5 + i, "G") = "Login Error"
Sheets("Clients").Cells(5 + i, "H") = "Login Error"
End If
Next
End Sub
Sub AlgomojoMulitOrder()
Dim Broker As String
Dim Version As String
Dim ClientID As String
Dim user_apikey As String
Dim api_secret As String
Dim masterapikey As String
Dim masterapisecret As String
Dim s_prdt_ali As String
Dim tsym As String
Dim exch As String
Dim trantype As String
Dim ret As String
Dim prctyp As String
Dim qty As String
Dim dscqty As String
Dim MktPro As String
Dim prc As String
Dim trgprc As String
Dim prd As String
Dim AMO As String
Dim ordersource As String
Dim remarks As String
Dim MultiplierQty As Long
Dim apidata As String
Dim numofRows As Long
Dim i As Integer
s_prdt_ali = "BO:BO||CNC:CNC||CO:CO||MIS:MIS||NRML:NRML"
MktPro = "NA"
dscqty = "0"
AMO = "NO"
ordersource = "WEB"
remarks = "EXCEL"
Broker = Sheets("PlaceOrder").Cells(5, "A").Value
Version = Sheets("PlaceOrder").Cells(5, "B").Value
masterapikey = Sheets("PlaceOrder").Cells(5, "D").Value
masterapisecret = Sheets("PlaceOrder").Cells(5, "E").Value
tsym = Sheets("PlaceOrder").Cells(5, "F").Value
qty = Sheets("PlaceOrder").Cells(5, "G").Value
trantype = Sheets("PlaceOrder").Cells(5, "H").Value
exch = Sheets("PlaceOrder").Cells(5, "I").Value
prd = Sheets("PlaceOrder").Cells(5, "J").Value
prctyp = Sheets("PlaceOrder").Cells(5, "K").Value
ret = Sheets("PlaceOrder").Cells(5, "L").Value
prc = Sheets("PlaceOrder").Cells(5, "M").Value
trgprc = Sheets("PlaceOrder").Cells(5, "N").Value
dscqty = Sheets("PlaceOrder").Cells(5, "O").Value
'clear the table contents of the display output
Worksheets("PlaceOrder").Range("C16:F200").Clear
Sheets("Clients").Activate
numofRows = Sheets("Clients").Range("A5", Range("A5").End(xlDown)).Rows.Count
apidata = "{""" & "orders" & """:" & "[ "
stgy_name = "Excel"
For i = 0 To numofRows - 1
Sheets("Clients").Activate
ClientID = Sheets("Clients").Cells(5 + i, "B").Value
user_apikey = Sheets("Clients").Cells(5 + i, "C").Value
api_secret = Sheets("Clients").Cells(5 + i, "D").Value
MultiplierQty = Sheets("Clients").Cells(5 + i, "E").Value * CInt(qty)
Sheets("PlaceOrder").Activate
Sheets("PlaceOrder").Cells(16 + i, "C") = ClientID
apidata = apidata & "{""" _
& "order_refno" & """:""" & CStr(i + 1) & """,""" _
& "user_apikey" & """:""" & user_apikey & """,""" _
& "api_secret" & """:""" & api_secret & """,""" _
& "stgy_name" & """:""" & stgy_name & """,""" _
& "uid" & """:""" & ClientID & """,""" _
& "actid" & """:""" & ClientID & """,""" _
& "tsym" & """:""" & tsym & """,""" _
& "exch" & """:""" & exch & """,""" _
& "trantype" & """:""" & trantype & """,""" _
& "ret" & """:""" & ret & """,""" _
& "prctyp" & """:""" & prctyp & """,""" _
& "qty" & """:""" & CStr(MultiplierQty) & """,""" _
& "dscqty" & """:""" & dscqty & """,""" _
& "MktPro" & """:""" & MktPro & """,""" _
& "prc" & """:""" & prc & """,""" _
& "prd" & """:""" & prd & """,""" _
& "AMO" & """:""" & AMO & """,""" _
& "ordersource" & """:""" & ordersource & """,""" _
& "remarks" & """:""" & remarks
If i = numofRows - 1 Then
apidata = apidata & """}"
Else
apidata = apidata & """},"
End If
Next
apidata = apidata & "]}"
'MsgBox (apidata)
PlaceOrder = AMConnect(masterapikey, masterapisecret, Broker, Version, "PlaceMultiOrder", apidata)
Set PlaceOrderResponse = JsonConverter.ParseJson(PlaceOrder)
For i = 0 To numofRows - 1
If StrComp(PlaceOrderResponse(i + 1)("stat"), "Ok") = 0 Then
Sheets("PlaceOrder").Cells(16 + i, "D").Value = PlaceOrderResponse(i + 1)("stat")
Sheets("PlaceOrder").Cells(16 + i, "E").Value = tsym
Sheets("PlaceOrder").Cells(16 + i, "F").Value = PlaceOrderResponse(i + 1)("norenordno")
Sheets("PlaceOrder").Cells(16 + i, "F").NumberFormat = "0"
Else
Sheets("PlaceOrder").Cells(16 + i, "D") = "Login Error"
Sheets("PlaceOrder").Cells(16 + i, "E") = "Login Error"
Sheets("PlaceOrder").Cells(16 + i, "F") = "Login Error"
End If
Next
'check multiorder response
'Sheets("PlaceOrder").Cells(12, "D") = PlaceOrder
End Sub
Private Function AMConnect(api_key As String, api_secret As String, Broker As String, Version As String, api_name As String, InTD As String) As String
Dim objHTTP As Object
Dim result As String
Dim postdate As String
Dim BrkPrefix As String
Dim BaseURL As String
BaseURL = "https://" & LCase(Broker) & "api.algomojo.com/" & Version & "/"
postdata = "{""" & "api_key" & """:""" & api_key & """,""" _
& "api_secret" & """:""" & api_secret & """,""" _
& "data" & """:" & InTD & "}"
'MsgBox (postdata)
Set objHTTP = CreateObject("MSXML2.ServerXMLHTTP")
Url = BaseURL & api_name
objHTTP.Open "POST", Url, False
objHTTP.setRequestHeader "Content-type", "application/json"
objHTTP.send (postdata)
result = objHTTP.responseText
Set objHTTP = Nothing
AMConnect = result
End Function
Credits
VBA Code uses VBA-JSON for Json Processing
hi,
can we use this excel file with some other broker.
i had trading account in upstox…
Hi,
Can we place FNO order for FIRSTOCK through excel.
sir, i am having setups to implement from trading view. having trading view premium subscription. can you team extend support to create algo
Can We get it for Finvasia?